Avatar
Sizes
Select size from 6 | 8 | 12 | 24 | 48.
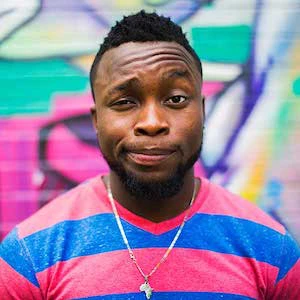
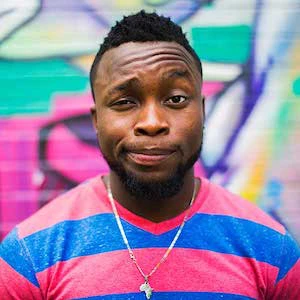
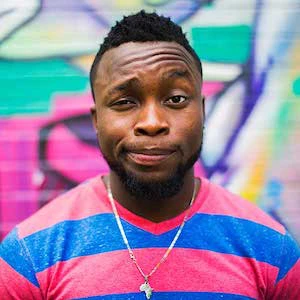
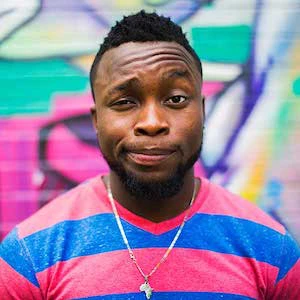
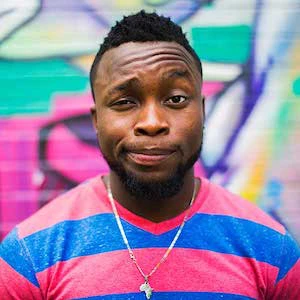
<script>
import { Avatar } from "$lib/index"
let avatar6 = {
src: '/images/profile-picture-1.webp',
alt: 'My avatar',
size: 6
}
let avatar8= {
src: '/images/profile-picture-1.webp',
alt: 'My avatar',
size: 8
}
let avatar12 = {
src: '/images/profile-picture-1.webp',
alt: 'My avatar',
size: 12
}
let avatar24 = {
src: '/images/profile-picture-1.webp',
alt: 'My avatar',
size: 24
}
let avatar48 = {
src: '/images/profile-picture-1.webp',
alt: 'My avatar',
size: 48,
}
</script>
<Avatar avatar={avatar6} />
<Avatar avatar={avatar8}/>
<Avatar avatar={avatar12}/>
<Avatar avatar={avatar24}/>
<Avatar avatar={avatar48}/>
Border and round
Set true to border and/or round.
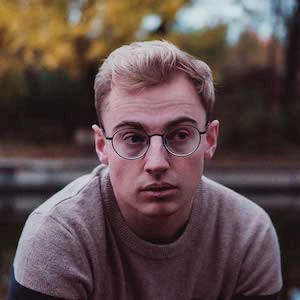
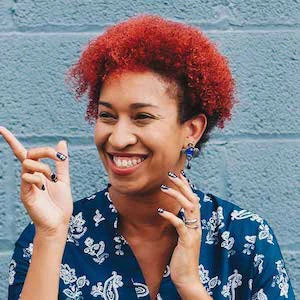
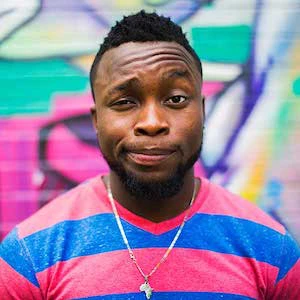
<script>
let avatar2 = {
src: '/images/profile-picture-2.webp',
alt: 'My avatar 2',
size: 12,
round: true
}
let avatar3 = {
src: '/images/profile-picture-3.webp',
alt: 'My avatar 3',
size: 12,
border: true,
}
let avatar4 = {
src: '/images/profile-picture-1.webp',
alt: 'My avatar 4',
size: 12,
border: true,
round: true
}
</script>
<Avatar avatar={avatar2} />
<Avatar avatar={avatar3} />
<Avatar avatar={avatar4} />
Placeholder
By setting placehoder, you display a placeholder avatar.
<Avatar placehoder />
Avatar text
You can set header and text to show additional information.
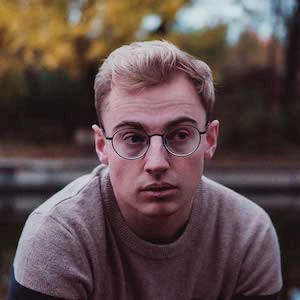
Jese Leos
Joined in August 2014
<script>
let avatarText ={
src: '/images/profile-picture-2.webp',
alt: 'My avatar 4',
size: 12,
border: true,
round: true,
header:'Jese Leos',
text:'Joined in August 2014'
}
</script>
<Avatar avatar={avatarText} />
Props
The component has the following props, type, and default values. See type-list page for type information.
Name | Type | Default |
---|---|---|
avatar | AvatarType | {} |
avatarClass | string | `w-${avatar.size} h-${avatar.size} ${isCircle} ${isBorder}` |
placehoder | boolean | false |